Welcome to our comprehensive guide on MERN stack technical interview questions! Mastering the MERN stack—MongoDB, Express.js, React, and Node.js—has become a cornerstone for developers venturing into modern web development. Aspiring developers and seasoned professionals alike are constantly seeking ways to showcase their proficiency in these technologies, especially when preparing for technical interviews.
We will look at curated list of interview questions tailored specifically to the MERN stack. Whether you’re a fresh graduate aiming to break into the industry or a seasoned developer looking to expand your skill set, these questions will help you navigate the intricacies of MERN stack technologies and ace your technical interviews.
List of Mern Stack Technical Interview Questions
Below is list of mern stack technical interview questions that will
MongoDB:
- What is the primary purpose of MongoDB in a MERN stack?
- Discuss MongoDB’s role as a NoSQL database, emphasizing its scalability and flexibility in handling diverse data types for web applications.
- Explain the concept of collections and documents in MongoDB.
- Define collections as containers for documents and elaborate on documents as individual records within a collection, highlighting their JSON-like structure.
- How does MongoDB handle relationships between data?
- Discuss MongoDB’s approaches to relationships, including embedding documents, referencing, and denormalization, and their impact on performance and scalability.
- Discuss the indexing strategies in MongoDB and their significance.
- Explain the importance of indexing for query performance, covering various types of indexes (e.g., single-field, compound) and scenarios where indexing is beneficial.
- Explain the aggregation framework in MongoDB and its use cases.
- Detail the aggregation pipeline’s stages and operators, demonstrating how it facilitates data processing and analysis within MongoDB.
- What are the advantages and disadvantages of using MongoDB?
- List and discuss MongoDB’s strengths, such as schema flexibility, horizontal scaling, and its document-oriented nature, along with potential drawbacks like lack of ACID compliance in certain scenarios.
- How does MongoDB ensure high availability and scalability?
- Explain MongoDB’s replication and sharding mechanisms, emphasizing how they ensure fault tolerance and horizontal scalability.
- Explain the difference between MongoDB’s WiredTiger and MMAPv1 storage engines.
- Contrast the features and performance characteristics of these storage engines in MongoDB, highlighting their strengths and use cases.
- Discuss the ACID properties in the context of MongoDB.
- Explain how MongoDB supports ACID properties and in which scenarios it ensures data consistency, isolation, durability, and atomicity.
- Explain the process of sharding in MongoDB and its benefits.
- Detail the sharding process in MongoDB, covering shard keys, chunks, and the advantages it offers for horizontal scaling.
- How does MongoDB handle transactions?
- Explain the support for multi-document transactions in MongoDB, detailing their usage, limitations, and performance considerations.
- Describe the concept of capped collections in MongoDB.
- Discuss capped collections as fixed-size collections with a circular structure, highlighting their use cases for logging or maintaining a limited history of data.
- What are TTL indexes in MongoDB, and how are they used?
- Explain Time-To-Live indexes, their purpose in MongoDB for automatic document expiration, and scenarios where they are beneficial.
- Discuss the security measures available in MongoDB.
- Cover authentication, role-based access control, encryption, and other security features available in MongoDB for safeguarding data.
- How can you optimize MongoDB queries for better performance?
- Discuss query optimization techniques such as index optimization, query profiling, aggregation pipeline optimization, and effective use of query hints.
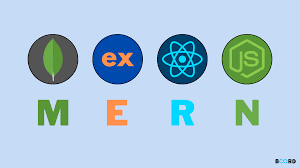
Express.js:
- What role does Express.js play in a MERN stack?
- Discuss Express.js as a minimalist web application framework for Node.js, enabling the development of robust and scalable server-side applications.
- Explain the core features of Express.js.
- Detail features like routing, middleware support, template engines, and HTTP utility methods provided by Express.js for building web applications.
- How does Express handle routing and why is it important?
- Explain Express’s routing mechanism for mapping HTTP requests to specific endpoints, emphasizing its role in organizing application logic and handling different request types.
- Describe the use of middleware in an Express.js application.
- Elaborate on middleware functions and their role in intercepting and modifying incoming requests or responses, enabling tasks like authentication, logging, or error handling.
- How can you handle file uploads in Express.js?
- Explain how to use middleware like ‘multer’ in Express.js for handling file uploads, specifying file size limits, handling different file types, and storing uploaded files.
- Discuss the differences between app.use() and app.get() in Express.js.
- Contrast app.use() as a middleware registration method and app.get() as a method for handling GET requests, explaining their respective uses and syntax.
- Explain error handling mechanisms in Express.js middleware.
- Describe approaches for error handling, such as using synchronous and asynchronous middleware functions, the ‘next’ function, and the ‘error’ object.
- How does Express.js handle sessions and cookies?
- Discuss session management and cookies in Express.js, covering middleware like ‘express-session’ for handling sessions and ‘cookie-parser’ for parsing cookies.
- Discuss the concept of template engines in Express.js.
- Explain the purpose of template engines like EJS or Handlebars in Express.js for server-side rendering of dynamic content.
- What is the purpose of route parameters in Express.js?
- Explain how route parameters are used to capture values from URL segments in Express.js and how they facilitate dynamic routing and handling of user input.
- Explain the concept of static files in Express.js and how to serve them.
- Detail the use of Express.js to serve static files such as images, CSS, and client-side JavaScript, explaining the ‘express.static()’ middleware.
- How can you implement authentication using Passport.js with Express?
- Discuss the integration of Passport.js, covering authentication strategies like local, OAuth, or JWT, and how they’re implemented with Express.js middleware.
- Discuss Express.js support for WebSockets.
- Explain how libraries like ‘socket.io’ can be integrated into Express.js for real-time bidirectional communication between the client and server.
- Explain how to perform unit testing in an Express.js application.
- Discuss testing tools like Mocha, Chai, or Jest for writing and executing unit tests in Express.js applications, covering mocking, stubbing, and assertions.
- How can you optimize Express.js applications for performance?
- Discuss performance optimization techniques such as caching, gzip compression, load balancing, and minimizing blocking code for enhanced application performance.
Check on Comparison of MERN Tech Stack with Tech Stack
Conclusion
In conclusion, the MERN stack technical interview questions covered in this guide provide a holistic view of what to expect when facing interviews for roles involving MongoDB, Express.js, React, and Node.js. Mastering these technologies goes beyond rote memorization of concepts; it’s about understanding their nuances and applying them effectively to real-world scenarios.
Remember, technical interviews aren’t just about showcasing knowledge; they’re an opportunity to exhibit problem-solving skills, logical thinking, and the ability to adapt these technologies to diverse challenges. Continuously practicing these interview questions, understanding the underlying concepts, and experimenting with hands-on projects will undoubtedly enhance your confidence and proficiency in the MERN stack.
We hope this guide serves as a valuable resource in your journey toward acing MERN stack technical interviews. Keep learning, stay curious, and embrace the ever-evolving landscape of web development with the MERN stack!