computer science interview questions
Welcome to our computer science interview! In this session, we aim to delve into your knowledge, experience, and problem-solving skills within the dynamic realm of computer science. This interview is designed to explore various facets of your expertise, from theoretical foundations to practical applications.
Through a series of thought-provoking questions, we seek to understand your approach to challenges, your capacity for innovation, and your ability to adapt in this ever-evolving field. We encourage you to showcase your unique perspective, insights, and passion for computer science as we embark on this exploration together.
The following are computer science interview questions that you can encounter when you are invited for a job interview. Our client will need to go for job interviews. After completion of IT /computer science course. We have provided some tips for that.
Computer Science Interview Questions
- Algorithm Design: Explain the concept of a greedy algorithm. Provide an example where a greedy approach might not yield the most optimal solution.
- Data Structures and Algorithms:
- Explain the difference between an array and a linked list.
- Describe the concept of Big O notation and its significance in algorithm analysis.
- What are the differences between an array and a linked list? In which scenarios would you choose one over the other?
- How would you implement a binary search tree? Can you explain its operations?
- Programming Languages and Concepts:
- What are the differences between object-oriented programming and functional programming?
- Discuss the importance of polymorphism and inheritance in object-oriented programming.
- Explain the concept of recursion and provide an example.
- Operating Systems:
- What is the role of an operating system? Name different types of operating systems.
- Describe the difference between process and thread in an operating system.
- How does virtual memory work, and what are its benefits?
- Databases:
- What is normalization in databases? Why is it important?
- Explain the differences between SQL and NoSQL databases.
- How would you optimize a slow-performing database query?
- Discuss the differences between SQL and NoSQL databases. In what scenarios would you choose one over the other for a project?
- Software Development and Engineering:
- Discuss the Software Development Life Cycle (SDLC) phases.
- How do you approach debugging and troubleshooting in a software project?
- Explain the importance of version control systems like Git in software development.
- Cybersecurity:
- What are some common cybersecurity threats, and how would you mitigate them?
- Describe the differences between symmetric and asymmetric encryption.
- How does HTTPS ensure secure communication over the internet?
- Networking:
- Explain the OSI model and its different layers.
- Describe the difference between TCP and UDP protocols.
- How does DNS (Domain Name System) work?
- Object-Oriented Programming: Describe the principles of encapsulation, inheritance, and polymorphism in object-oriented programming. Provide examples to illustrate each concept.
- Operating Systems: Explain the difference between process and thread in the context of an operating system. What are the advantages and disadvantages of multithreading?
- Networks: What is the OSI model? Describe each layer briefly and provide examples of protocols that operate at each layer.
- Software Development: What is the importance of version control in software development? Explain how Git facilitates collaborative coding and branching strategies.
- Artificial Intelligence: Explain the difference between supervised and unsupervised learning in machine learning. Give examples of real-world applications for each.
- Cyber Security: What are some common types of cyber attacks on web applications, and how can they be mitigated or prevented?
- Coding Challenge: Provide an algorithm to find the shortest path in a graph using Dijkstra’s algorithm. Write pseudo-code or explain the steps involved.
- What types of projects have you worked on? (Clear and specific examples with elaboration)
- Can you walk me through a specific project you worked on? What was your role? Who were the stakeholders? What problem were you solving? What was the outcome?
- Work from home has become the new normal in the post-COVID-19-world. How well are you prepared to manage a remote team? How would you provide training if needed remotely?
- Tell us about the most challenging projects you have managed so far? What were the steps you have taken to tackle the challenges?
- What are the techniques you use to define the scope of a project?
- escribe the team forming process you follow in project management.
- Tell me about a team member that you helped grow in his role. How did you help him/her? (specific example)
- What are the three key challenges for our industry today (IT industry), and how do you think these can be tackled effectively?
- What are some examples of times you’ve accommodated additional requests from customers/stakeholders even when that might have been difficult?
- What are some best practices you’ve used to develop excellent customer relationships?
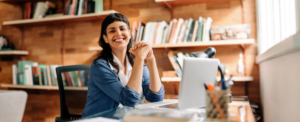
Additional Interview Questions
- Give a few examples of proactive decision-making in your past projects and your life in general.
- What experience do you have with process development? Tell me about a process or processes you’ve developed or helped develop. (specific examples)
- How do you motivate people to stay on track and meet their deadline? What would you say is the most difficult/challenging aspect of Project Management?
- Tell me about a time you had to learn to use a new software or tool. How did you go about it? (specific example)
- How do you ensure your projects are delivered on time and within budget?
- How do you handle missed deadlines?
- Tell me about a time you had to escalate the situation to upper management? (specific example)
- Tell me about your most successful project. (specific example)
Tell me about a time you experienced failure in your project management role. Tell me about a project
- How do you manage multiple projects at the same time? What are your techniques?
- How do you prioritize your workload?
- How do you manage risks in a project?
- How do you deal with changes in a project?
- Tell me how you manage a project from start to finish (Step by step)
Above are interview questions asked in computer science job interviews.
Read on Computer Science Assignment Help
Conclusion